React에서 .tsx 없이 Typescript 사용하기
2019.05.11(7달 전)
이번 글에서는 기존에 .js, .jsx 확장자로 작성된 React 컴포넌트에서 Typescript를 적용하여 컴포넌트 개발이나 사용 시에 타입을 확인할 수 있는 방법에 대해서 설명하려고 한다.
Button.js
컴포넌트로 간단한 예를 들어본다.
src/
components/
button/
index.js
Button.js
구조로 컴포넌트가 작성되어 있다고한다면. 동일한 파일 경로에 index.d.ts
를 만들자.
src/
components/
button/
index.js
+ index.d.ts
Button.js
index.d.ts
에는 button
에 필요한 Props
들을 Typescript로 작성한다.
export interface ButtonProps extends React.HTMLAttributes<HTMLButtonElement> {}
export default class Button extends React.Component<ButtonProps> {}
이 예제에서는 별다른 Props가 필요없이 Button 컴포넌트의 Typescript를 작성하는데 중점을 둔다.
그럼 이제 Button.js
를 작성한다.
import React, { Component } from 'react';
/**
* @augments {Component<import('.').ButtonProps>}
*/
class Button extends Component {
render() {
const { children, ...other } = this.props;
return <button {...other}>{children}</button>;
}
}
export default Button;
이렇게 작성된 Button.js
컴포넌트의 this.props
의 deconstructing 부분에서 ctrl + space
로 힌트를 쳐보면 다음과 같이 제공할 수 있는 props들이 힌트로 나오게된다.
이제 이 Button.js
컴포넌트를 가지고 다른 컴포넌트에서 사용해본다.
추가적으로 functional component
에서는 다음처럼 작성할 수 있다.
import React, { Component } from 'react';
/**
* @description
* @param {import('.').ButtonProps} props
* @returns {React.ReactNode}
*/
const Button = (props) => {
const { children, ...other } = props;
return (
<button {...other}>{children}</button>
)
}
export default Button;
힌트도 똑같이 사용할 수 있다.
react
typescript
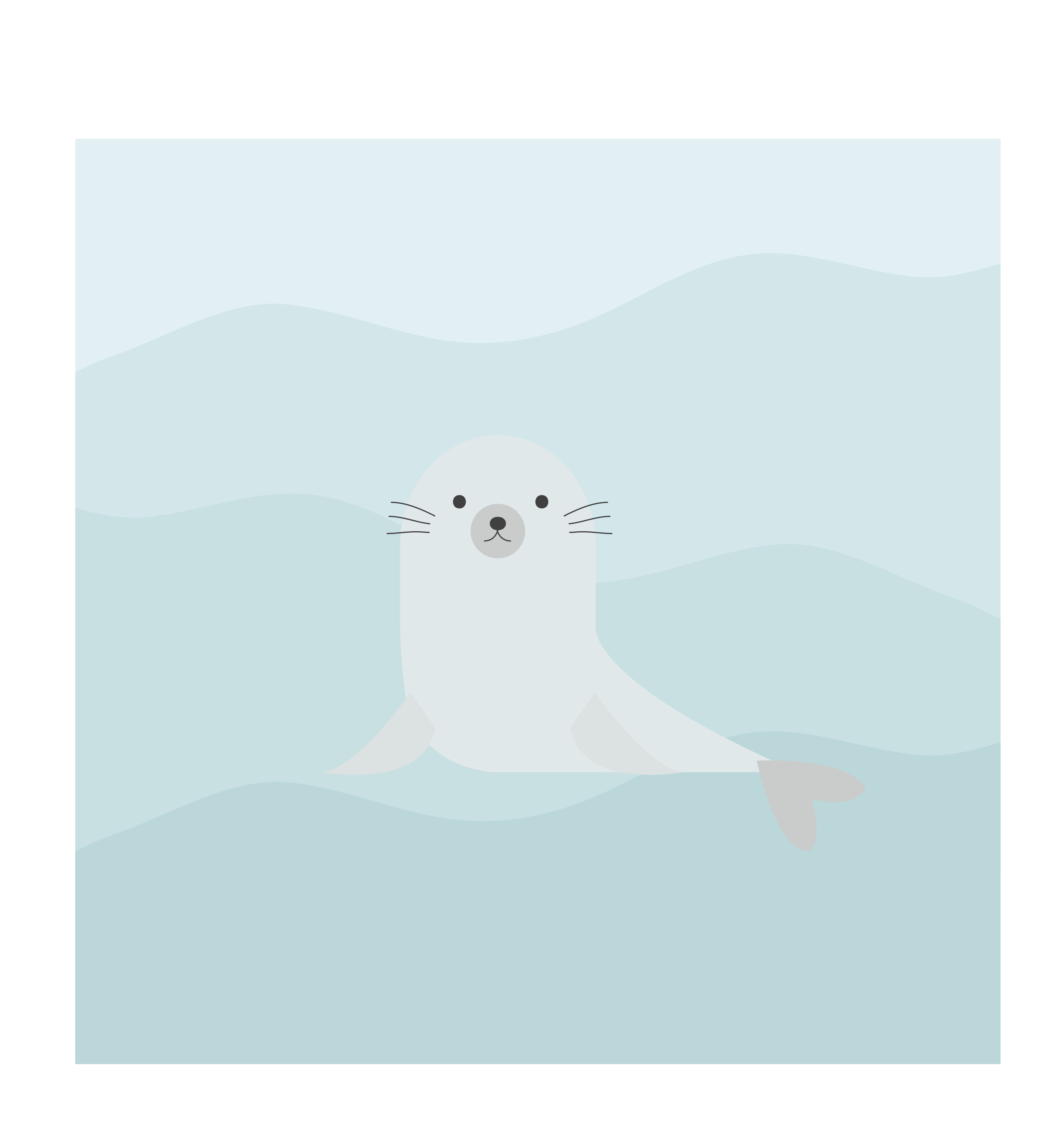
Sung Gyun Oh
Hello world!